In this tutorial, we will learn how to use a for loop in Alpine.js. We will cover the x-for
loop with numbers, looping through arrays, x-for
loop index iteration, and looping through associative arrays (key-value pairs) using AlpineJS 3.
Example 1
Alpine.js Simple x-for
Loop Using Numbers.
<ul x-data="{ numbers: [1, 2, 3] }">
<template x-for="number in numbers">
<li x-text="number"></li>
</template>
</ul>
Example 2
Alpinejs x-for loop index iteration.
<div x-data="{ brands: ['Amazon', 'Apple', 'Google','Microsoft'] }">
<template x-for="(brand, index) in brands">
<div><span x-text="index"></span>:<span x-text="brand"></span></div>
</template>
</div>

Example 3
Alpinejs for loop with Iterating over a range.
<ul x-data>
<template x-for="i in 10">
<li x-text="i"></li>
</template>
</ul>
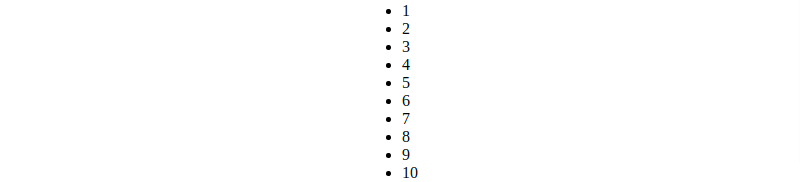
Example 4
Alpinejs x-for loop with associative arrays key value pair.
<ul x-data="{ people: [
{ id: 1, name: 'Jhon' },
{ id: 2, name: 'nike' },
{ id: 3, name: 'sam' },
]}">
<template x-for="person in people" :key="person.id">
<li x-text="person.name"></li>
</template>
</ul>
You can also clean alpinejs code to writer in bottom.
<ul x-data="employee">
<template x-for="person in people" :key="person.id">
<li x-text="person.name"></li>
</template>
</ul>
<script>
document.addEventListener('alpine:init', () => {
Alpine.data('employee', () => ({
people: [
{ id: 1, name: 'Jhon' },
{ id: 2, name: 'nike' },
{ id: 3, name: 'sam' },
],
}));
});
</script>
